JS笔记6:内建对象
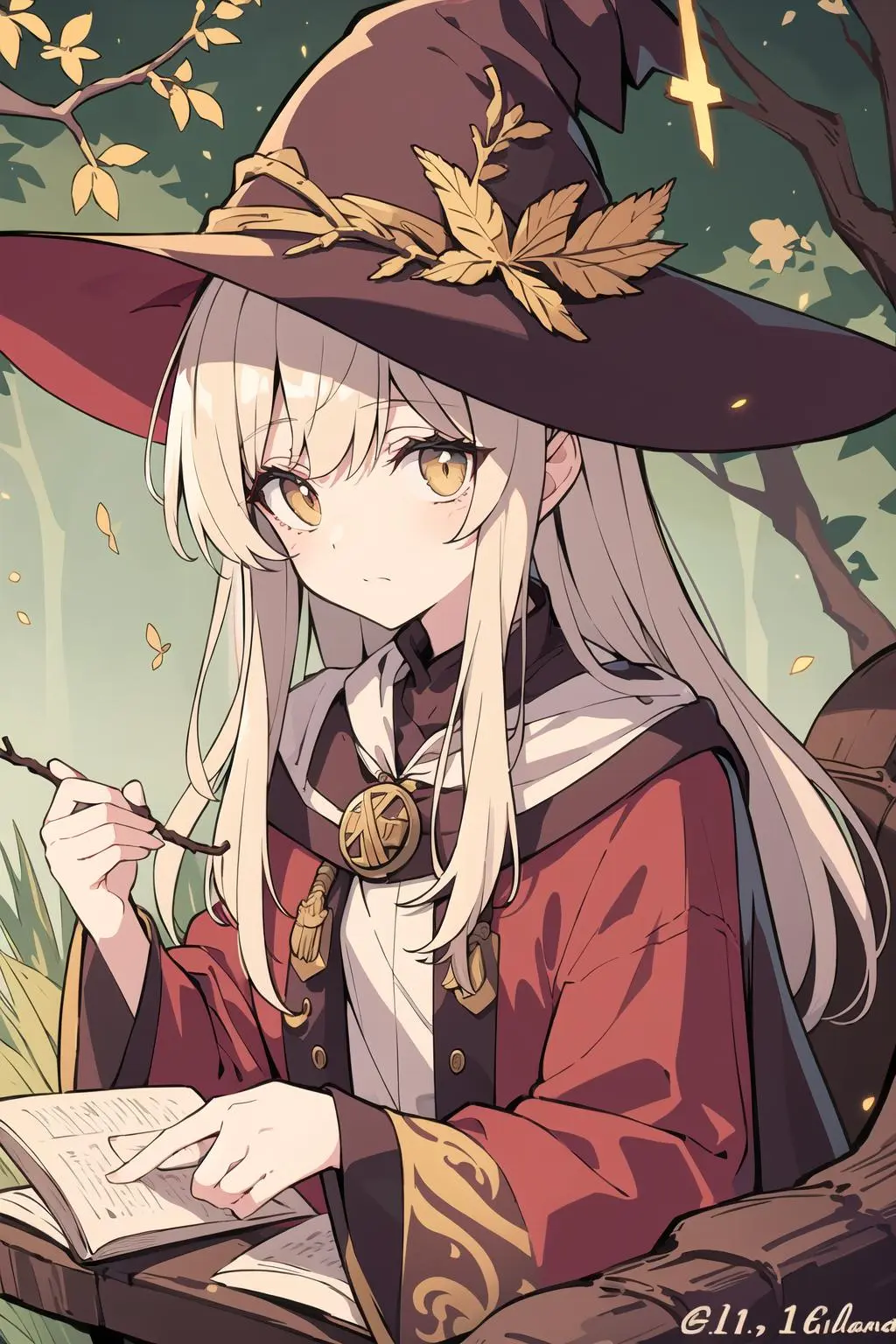
JS的常用对象包括 Array、Date、Math、String 等
- 数组 Array 中可以放任何类型的数据
1 | let arr = new Array(); |
push() 在末尾添加一个或多个元素并返回新的长度
pop() 删除并返回最后一个元素
unshift() 在开头添加一个或多个元素并返回新的长度
shift() 删除并返回第一个元素
1 | let arr = new Array(); // 2 |
数组的 forEach() 方法,需要传入一个函数对象,浏览器将各个元素的 currentValue, index, arr 作为实参传入函数并执行
IE8 及以下不支持
1
2
3
4
5
6
7
8let arr = ["hello", true];
arr.forEach(function(currentValue, index, arr) {
console.log(currentValue, index, arr); // 其中 arr 就是当前的数组
});
/*
* hello 0 Array [ "hello", true ]
* true 1 Array [ "hello", true ]
*/slice(start, end) 从某个已有的数组返回选定的元素,闭区间,end 参数可选,支持负数
1
2
3
4let arr = ["hello", true, null, 32];
console.log(arr.slice(0,2)); // Array [ "hello", true ]
console.log(arr.slice(2)); // Array [ null, 32 ]
console.log(arr.slice(-3)); // Array(3) [ true, null, 32 ]splice(start,howmany,item1…..) 删除原数组中指定数量的元素,添加新元素,并将删除的元素作为新数组返回
1
2
3let arr = ["hello", true, null, 32, new Person()];
arr.splice(2,3,"item1",666); // Array(3) [ null, 32, {} ]
console.log(arr); // Array(4) [ "hello", true, "item1", 666 ]concat() 连接多个数组作为新数组返回,不会改变现有的数组
1
2
3let arr1 = ["hello"], arr2 = [12];
console.log(arr1.concat(arr2)); // Array [ "hello", 12 ]
console.log(arr1); // Array [ "hello" ]join() 将数组的所有元素放入一个字符串,并用指定的分隔符进行分割(默认为逗号)
1 | let arr = [12, "hello"]; |
- reverse() 翻转原数组
1 | let arr = [12, "hello"]; |
- sort() 对原数组进行排序,可传入一个比较函数。需要升序排列则返回 a-b
1 | let arr = [40, 100, 1, 5, 25, 10]; |
- 直接使用构造函数创建一个 Date 对象,则会封装当前代码执行时间
1
2
3let d1 = new Date();
let d2 = new Date("12/05/2018 11:22:33");
console.log(d2); // Date Wed Dec 05 2018 11:22:33 GMT+0800 (中国标准时间)
Math 作为对象使用调用其属性和方法
1 | Math.ceil() , Math.floor() // 向上取整和向下取整 |
字符串是以字符数组保存的
字符串的大多数方法不改变原字符串
1 | charAt(index) // 返回字符串中指定 index 位置的字符。 |